Date |
Weight (lb) |
Fat (%) |
EndHTML
# Loop through dates and pull measurements from them, or display
# a blank entry for that day.
my @weekday_weights = ();
my @weekday_fats = ();
my @all_weights = ();
my @all_fats = ();
my @weights = ();
my @fats = ();
while($date le $end_date) {
my $measure = $measures->date($date);
my $weight = undef;
my $fat = undef;
my $weekday = date_weekday($date);
if($measure) {
$weight = $measure->weight;
$fat = $measure->fat;
push @all_weights, $weight;
push @all_fats, $fat;
push @weights, $weight;
push @fats, $fat;
if(defined $weekday_weights[$weekday]) {
push @{$weekday_weights[$weekday]}, $weight;
} else {
$weekday_weights[$weekday] = [ $weight ];
}
if(defined $weekday_fats[$weekday]) {
push @{$weekday_fats[$weekday]}, $fat;
} else {
$weekday_fats[$weekday] = [ $fat ];
}
if($weight <= $min_weight) {
$weight = sprintf '%0.1f', $weight;
} elsif($weight >= $max_weight) {
$weight = sprintf '%0.1f', $weight;
} else {
$weight = sprintf '%0.1f', $weight;
}
if($fat <= $min_fat) {
$fat = sprintf '%0.1f', $fat;
} elsif($fat >= $max_fat) {
$fat = sprintf '%0.1f', $fat;
} else {
$fat = sprintf '%0.1f', $fat;
}
} else {
$weight = $fat = ' '
}
printf(<< ' EndHTML', $date, $weight, $fat);
%s |
%s |
%s |
EndHTML
my $tomorrow = date_tomorrow($date);
if($weekday == 6) {
printf(<< ' EndHTML', mean(\@weights), mean(\@fats));
Mean |
%0.1f |
%0.1f |
EndHTML
@weights = ();
@fats = ();
if($tomorrow le $end_date) {
print << ' EndHTML';
Date |
Weight (lb) |
Fat (%) |
EndHTML
}
}
$date = $tomorrow;
}
# Add code to not print table end if we just printed it
unless(date_weekday($date) == 0) {
print << ' EndHTML';
EndHTML
}
print << 'EndHTML';
| |
EndHTML
my $delta_weight = sprintf '%0.1f', 0.0 + sprintf '%0.1f',
$measures->date($dates[$#dates])->ewma_weight -
$measures->date($dates[0])->ewma_weight ;
my $delta_fat = sprintf '%0.1f', 0.0 + sprintf '%0.1f',
$measures->date($dates[$#dates])->ewma_fat -
$measures->date($dates[0])->ewma_fat ;
my $ewma_weight = sprintf '%0.1f',
$measures->date($dates[$#dates])->ewma_weight ;
my $ewma_fat = sprintf '%0.1f',
$measures->date($dates[$#dates])->ewma_fat ;
my $mean_weight = sprintf '%0.1f', $measures->mean_weight;
my $mean_fat = sprintf '%0.1f', $measures->mean_fat;
my $weight_spread = sprintf '%0.1f',
abs($measures->max_weight - $measures->min_weight);
my $fat_spread = sprintf '%0.1f',
abs($measures->max_fat - $measures->min_fat);
my $stddev_weight = sprintf '%0.1f', $measures->stddev_weight;
my $stddev_fat = sprintf '%0.1f', $measures->stddev_fat;
print << "EndHTML";
Statistics |
Measurement |
Weight (lb) |
Fat (%) |
Weighted Delta |
$delta_weight |
$delta_fat |
Weighted Average |
$ewma_weight |
$ewma_fat |
Mean |
$mean_weight |
$mean_fat |
Minimum |
$min_weight |
$min_fat |
Maximum |
$max_weight |
$max_fat |
Spread |
$weight_spread |
$fat_spread |
Standard Deviation |
$stddev_weight |
$stddev_fat |
Weekday Means |
Weekday |
Weight (lb) |
Fat (%) |
EndHTML
foreach(0 .. $#weekday_weights) {
my $weekday = ( qw( Sunday Monday Tuesday Wednesday
Thursday Friday Saturday) )[$_];
my $weight = mean($weekday_weights[$_]);
my $fat = mean($weekday_fats[$_]);
$weight = sprintf '%0.1f', $weight;
$fat = sprintf '%0.1f', $fat;
printf(<< ' EndHTML', $weekday, $weight, $fat);
%s |
%s |
%s |
EndHTML
}
print << 'EndHTML';
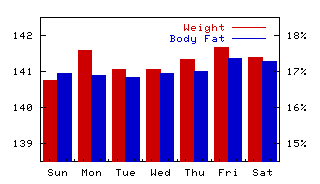 |
|